SGSDK Package Content
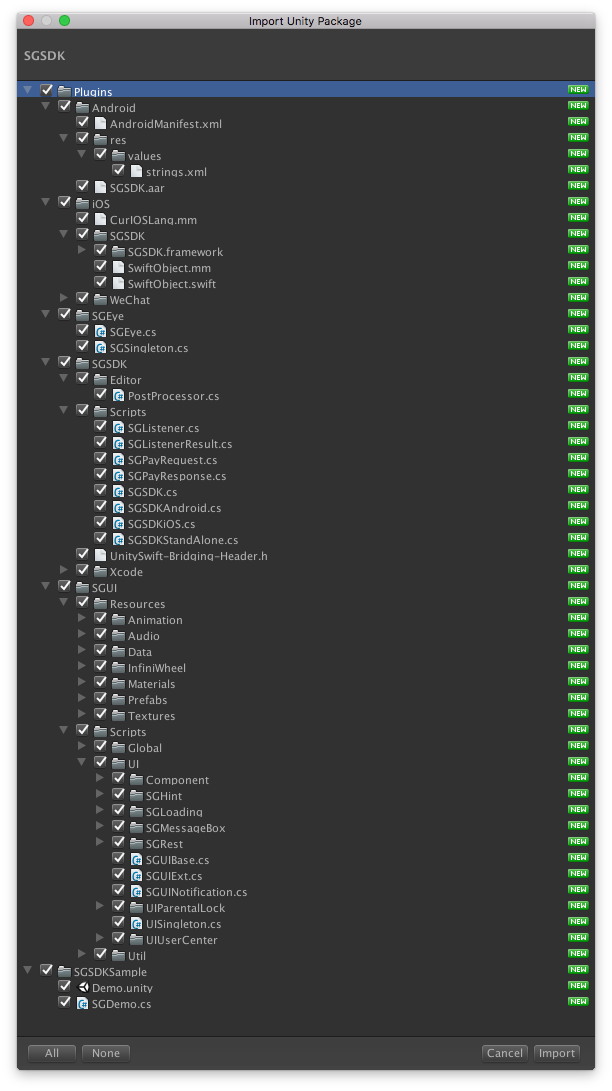
1.Android
- Plugins/Android/SGSDK.aar is native android sdk
- Plugins/Android/android-support-v4.jar is dependency sdk of android. Keeping the newest one avoid making apk error.
- Plugins/Android/AndroidManifest.xml is AndroidManifest.xml of sdk. Have to merge with project.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | <manifest xmlns:android="http://schemas.android.com/apk/res/android"> <application> <!-- SGSDK setting --> <!-- Launch SGUnityContext override from com.unity3d.player.UnityPlayerActivity --> <activity android:name="com.sgstudios.sdk.SGUnityActivity" android:launchMode="singleTask"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> <meta-data android:name="unityplayer.ForwardNativeEventsToDalvik" android:value="true" /> </activity> <!-- Enter channel key from https://apidocs.smartgamesltd.com/channel --> <meta-data android:name="SDKChannel" android:value="lemian" /> <!-- Google payment PublicKey --> <meta-data android:name="Base64PublicKey" android:value="MyPublicKey" /> <uses-sdk android:minSdkVersion="19" /> </application> </manifest> |
2.iOS
- Plugins/iOS/SGSDK.framework native iOS sdk
- Plugins/iOS/SwiftObject.mm SwiftObject.swift is intermediary file of iOS and Unity.
- Below are files of build iOS project necessary.
- Plugins/SGSDK/Editor/PostProcessor.cs
- Plugins/SGSDK/UnitySwift-Bridging-Header.h
- Plugins/SGSDK/Xcode/
3.Unity SDK
- Plugins/SGSDK/Scripts Unity SGSDK